Details
MINSTD is a Lehmer generator found in the C++ standard. This uses the revised constants proposed by Park and Miller in the 1980s, along with a more complex implementation that avoids 64-bit mathematics.
These changes make quite a large difference, as this generator passes nearly every test. Even the Plot test shows a decent distribution of outputs, though it could be better.
|
Pseudocode
// This code is adapted from MINSTD's implementation
// in the GNU Scientific Library.
//
// https://www.gnu.org/software/gsl/
// The current state value
state = 1138;
// Our constants
a = 16807;
M = 2147483647;
q = 127773;
r = 2836;
// random number generator
function RandomNumber() {
// Save the state
x = state;
// Run the math
h = int( x / q );
t = a * (x - (h * q)) - (h * r);
if (t < 0) {
t = t + M;
}
// Save the new value
state = t;
// And return it
return state;
}
|
Period Length TestSeed | Period Length | Result |
---|
1138 | 64000 | Passed | 65535 | 64000 | Passed | 8675309 | 64000 | Passed | 16777216 | 64000 | Passed | 123456789 | 64000 | Passed | Minimum to Pass: 64,000
| Plot Test 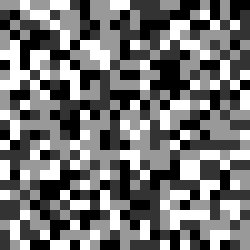 |
Count the 1s TestSeed | % Bits that are 1s | Result |
---|
1138 | 50.00% | Passed | 65535 | 49.97% | Passed | 8675309 | 50.14% | Passed | 16777216 | 49.83% | Passed | 123456789 | 49.83% | Passed | Minimum to Pass: 45%
| Dartboard TestSeed | Darts Placed | Result |
---|
1138 | 7014 | Passed | 65535 | 7000 | Passed | 8675309 | 6979 | Passed | 16777216 | 6969 | Passed | 123456789 | 6930 | Passed | Minimum to Pass: 2,600
|
Crush TestSeed | Compression Rate | Result |
---|
1138 | 101.14% | Passed | 65535 | 101.14% | Passed | 8675309 | 101.14% | Passed | 16777216 | 101.14% | Passed | 123456789 | 101.14% | Passed | Minimum to Pass: 95%
| Unique Bytes TestSeed | Unique High Bytes | Unique Low Bytes | Result |
---|
1138 | 167 | 165 | Passed | 65535 | 156 | 171 | Failed | 8675309 | 167 | 170 | Passed | 16777216 | 167 | 161 | Passed | 123456789 | 160 | 164 | Passed | Minimum to Pass: 160
|
High/Low Byte TestSeed | High After High | High After Low | Low After High | Low After Low | Spread | Result |
---|
1138 | 2523 | 2454 | 2454 | 2568 | 183 | Passed | 65535 | 2455 | 2490 | 2491 | 2563 | 127 | Passed | 8675309 | 2506 | 2502 | 2502 | 2489 | 21 | Passed | 16777216 | 2531 | 2510 | 2510 | 2448 | 103 | Passed | 123456789 | 2522 | 2490 | 2490 | 2497 | 45 | Passed | Maximum to Pass: 500
|
Distribution TestSeed | 1138 | 65535 | 8675309 | 16777216 | 123456789 |
---|
0.0 to 0.1 | 999 | 1011 | 1011 | 1010 | 1016 |
---|
0.1 to 0.2 | 988 | 971 | 970 | 1005 | 986 |
---|
0.2 to 0.3 | 1011 | 1007 | 1029 | 990 | 1068 |
---|
0.3 to 0.4 | 1025 | 986 | 1018 | 1015 | 969 |
---|
0.4 to 0.5 | 955 | 972 | 980 | 1022 | 975 |
---|
0.5 to 0.6 | 1016 | 994 | 1015 | 1011 | 1007 |
---|
0.6 to 0.7 | 991 | 1058 | 957 | 987 | 995 |
---|
0.7 to 0.8 | 1009 | 968 | 1036 | 973 | 967 |
---|
0.8 to 0.9 | 1010 | 1022 | 1034 | 1027 | 1004 |
---|
0.9 to 1.0 | 997 | 1012 | 951 | 961 | 1014 |
---|
Spread | 141 | 219 | 285 | 179 | 217 |
---|
Result | Passed | Passed | Passed | Passed | Passed |
---|
Maximum to Pass: 500 |
Sample Output1924514014 | 2055825831 | 1400345034 | 1325698965 | 879667130 | 1288027962 | 1250795574 | 403791735 | 479365625 | 1486899478 | 52326607 | 1132472226 | 313139021 | 1592590797 | 437348971 | 1835115563 | 627129127 | 309498013 | 527711457 | 138995689 | 1785820734 | 1057625866 | 795783643 | 207534385 | 516965967 | 2075655254 | 1813492110 | 126490899 | 2071212610 | 160418400 | 1060071815 | 1102659193 | 1756666788 | 693526960 | 1713864451 | 721670746 | 132589766 | 1495655223 | 1181244826 | 1842957714 | 1433658517 | 732175879 | 598701043 | 1407543506 | 2051333637 | 1061968121 | 761619430 | 1535223890 | 491900525 | 1707566372 | 96555696 | 1461429187 | 1469875170 | 1687590749 | 1521192514 | 889765263 | 1356141180 | 1420866649 | 487615103 | 549439169 | 244431283 | 20356670 | 684652817 | 742514693 | 416972534 | 818238777 | 1801333298 | 1931767727 | 1562412343 | 34213285 | 1644547246 | 1791026632 | 506324025 | 1457678761 | 713491151 | 97090009 | 1851693190 | 74432006 | 1143242288 | 936944707 | 1879590745 | 797203845 | 454549282 | 1010450195 | 335746889 | 1458422754 | 332879620 | 512872905 | 2003038924 | 1121545296 | 1347820153 | 1155802915 | 1590005290 | 2079889409 | 2109974844 | 949740197 | 37542828 | 1769601625 | 1193484072 | 1389535124 |
|