Details
Doom quickly became a cult classic soon after its release in the mid-1990s. Surprisingly though, the way it handles random number generation is extremely primitive: the numbers are simply indexes in a hard-coded table of 256 values. The only calculation being performed is moving the pointer to the next index. This shockingly simple method works quite well, suggesting that perhaps this table was originally created using the output of a more advanced PRNG.
Another advantage of such a simple PRNG is that it makes syncing multiplayer games much easier, as their PRNGs would always return the same output. It also permitted players to make recordings of their adventure, as the random number rolls were guaranteed to be the same during playback.
However, the obvious limits to this design ensured that it would never pass the Period or Plot tests.
|
Pseudocode
// Adapted from the source at
// https://github.com/id-Software/DOOM
// The current state value
state = 0;
// PRNG table
values = [ ... ];
// random number generator
function RandomNumber() {
// Return the new value
return values[state];
// Move to the next slot
state++;
if (state >= count(values)) {
state = 0;
}
}
|
Period Length TestSeed | Period Length | Result |
---|
1138 | 258 | Failed | 65535 | 258 | Failed | 8675309 | 258 | Failed | 16777216 | 258 | Failed | 123456789 | 258 | Failed | Minimum to Pass: 64,000
| Plot Test 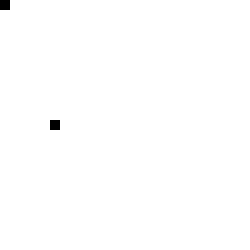 |
Count the 1s TestSeed | % Bits that are 1s | Result |
---|
1138 | 49.52% | Passed | 65535 | 49.52% | Passed | 8675309 | 49.53% | Passed | 16777216 | 49.50% | Passed | 123456789 | 49.51% | Passed | Minimum to Pass: 45%
| Dartboard TestSeed | Darts Placed | Result |
---|
1138 | 127 | Failed | 65535 | 128 | Failed | 8675309 | 128 | Failed | 16777216 | 126 | Failed | 123456789 | 128 | Failed | Minimum to Pass: 2,600
|
Crush TestSeed | Compression Rate | Result |
---|
1138 | 4.72% | Failed | 65535 | 4.73% | Failed | 8675309 | 4.74% | Failed | 16777216 | 4.73% | Failed | 123456789 | 4.73% | Failed | Minimum to Pass: 95%
| Unique Bytes TestSeed | Unique High Bytes | Unique Low Bytes | Result |
---|
1138 | 165 | 165 | Passed | 65535 | 165 | 165 | Passed | 8675309 | 165 | 165 | Passed | 16777216 | 165 | 165 | Passed | 123456789 | 165 | 165 | Passed | Minimum to Pass: 160
|
High/Low Byte TestSeed | High After High | High After Low | Low After High | Low After Low | Spread | Result |
---|
1138 | 2604 | 2442 | 2441 | 2512 | 233 | Passed | 65535 | 2592 | 2442 | 2443 | 2522 | 229 | Passed | 8675309 | 2593 | 2444 | 2444 | 2518 | 223 | Passed | 16777216 | 2591 | 2441 | 2442 | 2525 | 233 | Passed | 123456789 | 2595 | 2440 | 2440 | 2524 | 239 | Passed | Maximum to Pass: 500
|
Distribution TestSeed | 1138 | 65535 | 8675309 | 16777216 | 123456789 |
---|
0.0 to 0.1 | 888 | 886 | 887 | 889 | 889 |
---|
0.1 to 0.2 | 927 | 926 | 923 | 926 | 930 |
---|
0.2 to 0.3 | 1082 | 1081 | 1082 | 1081 | 1080 |
---|
0.3 to 0.4 | 1159 | 1159 | 1160 | 1158 | 1157 |
---|
0.4 to 0.5 | 969 | 962 | 966 | 962 | 963 |
---|
0.5 to 0.6 | 1039 | 1046 | 1042 | 1046 | 1042 |
---|
0.6 to 0.7 | 1004 | 1006 | 1009 | 1007 | 999 |
---|
0.7 to 0.8 | 1001 | 1006 | 1005 | 1006 | 1008 |
---|
0.8 to 0.9 | 850 | 848 | 847 | 848 | 853 |
---|
0.9 to 1.0 | 1004 | 1003 | 1002 | 1002 | 1004 |
---|
Spread | 655 | 679 | 677 | 675 | 657 |
---|
Result | Failed | Failed | Failed | Failed | Failed |
---|
Maximum to Pass: 500 |
Sample Output178 | 252 | 182 | 202 | 182 | 141 | 197 | 4 | 81 | 181 | 242 | 145 | 42 | 39 | 227 | 156 | 198 | 225 | 193 | 219 | 93 | 122 | 175 | 249 | 0 | 175 | 143 | 70 | 239 | 46 | 246 | 163 | 53 | 163 | 109 | 168 | 135 | 2 | 235 | 25 | 92 | 20 | 145 | 138 | 77 | 69 | 166 | 78 | 176 | 173 | 212 | 166 | 113 | 94 | 161 | 41 | 50 | 239 | 49 | 111 | 164 | 70 | 60 | 2 | 37 | 171 | 75 | 136 | 156 | 11 | 56 | 42 | 146 | 138 | 229 | 73 | 146 | 77 | 61 | 98 | 196 | 135 | 106 | 63 | 197 | 195 | 86 | 96 | 203 | 113 | 101 | 170 | 247 | 181 | 113 | 80 | 250 | 108 | 7 | 255 |
|